Pythonを使って指定のURLのフルスクリーンショットを保存する方法
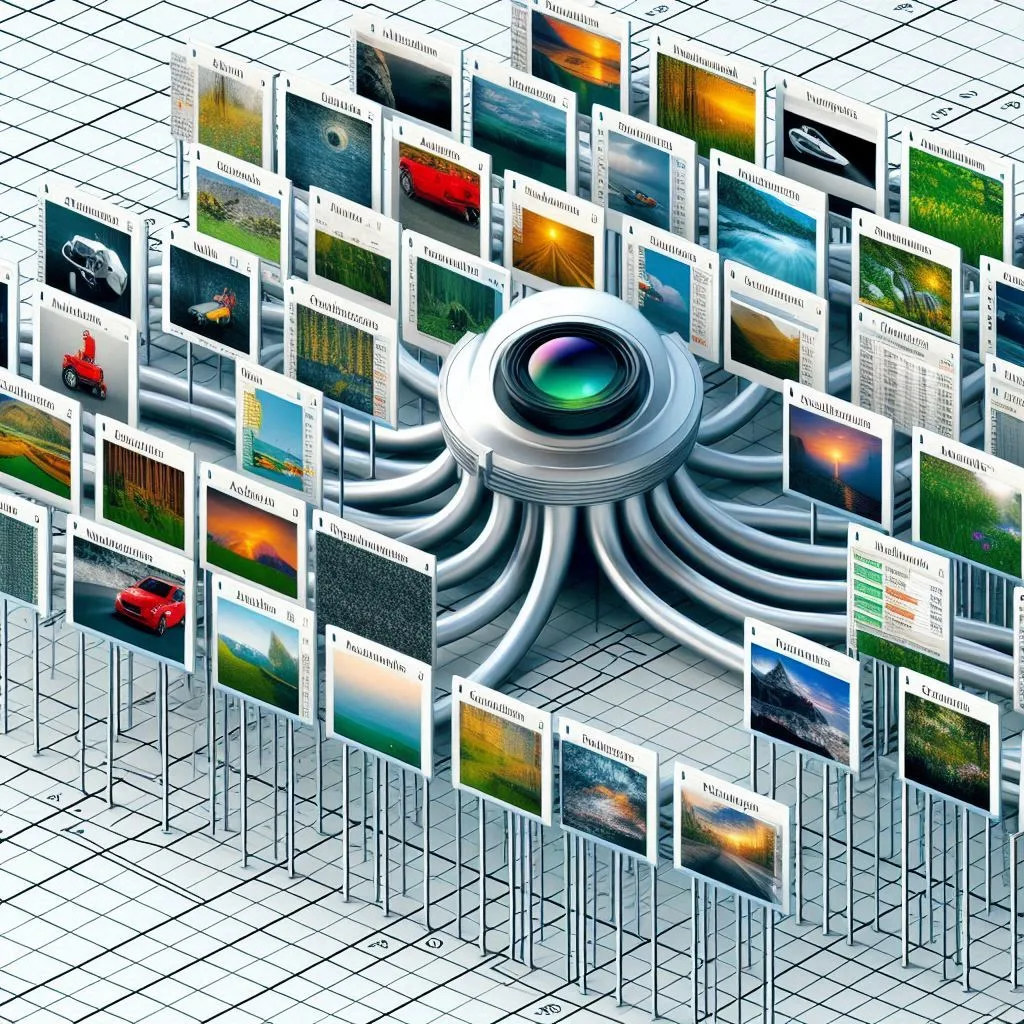
Web環境のPythonを使ってURLリストのフルスクリーンショットを保存する方法です。
この手順では、Seleniumを使用して各URLにアクセスし、
フルスクリーンのスクリーンショットを作成します。
windows は Pythonはインストールが必要なのでこのサイトを参考に設定
必要なライブラリのインストール
まず、必要なライブラリをインストールします。
以下のコマンドプロンプトを使用してSeleniumとWebドライバマネージャをインストールしてください。(windowsのコマンドプロンプト)
コマンドプロンプトを起動するには
- Windows キーと R キーを同時に押して「ファイル名を指定して実行」ダイアログを表示し、「cmd.exe」と入力します。
- スタートメニューから「Windows システムツール」→「コマンドプロンプト」を選択します。
- スタートメニューから「すべてのプログラム」→「アクセサリ」→「コマンドプロンプト」を選択します。
pip install selenium webdriver-manager
実行すると・・・
Microsoft Windows [Version 10.0.22621.4890]
(c) Microsoft Corporation. All rights reserved.
C:\Users\xxxxx>pip install selenium webdriver-manager
Requirement already satisfied: selenium in c:\data\python\lib\site-packages (4.29.0)
Collecting webdriver-manager
Downloading webdriver_manager-4.0.2-py2.py3-none-any.whl.metadata (12 kB)
Requirement already satisfied: urllib3<3,>=1.26 in c:\data\python\lib\site-packages (from urllib3[socks]<3,>=1.26->selenium) (2.3.0)
Requirement already satisfied: trio~=0.17 in c:\data\python\lib\site-packages (from selenium) (0.29.0)
Requirement already satisfied: trio-websocket~=0.9 in c:\data\python\lib\site-packages (from selenium) (0.12.2)
Requirement already satisfied: certifi>=2021.10.8 in c:\data\python\lib\site-packages (from selenium) (2025.1.31)
Requirement already satisfied: typing_extensions~=4.9 in c:\data\python\lib\site-packages (from selenium) (4.12.2)
Requirement already satisfied: websocket-client~=1.8 in c:\data\python\lib\site-packages (from selenium) (1.8.0)
Requirement already satisfied: requests in c:\data\python\lib\site-packages (from webdriver-manager) (2.32.3)
Collecting python-dotenv (from webdriver-manager)
Downloading python_dotenv-1.0.1-py3-none-any.whl.metadata (23 kB)
Collecting packaging (from webdriver-manager)
Downloading packaging-24.2-py3-none-any.whl.metadata (3.2 kB)
Requirement already satisfied: attrs>=23.2.0 in c:\data\python\lib\site-packages (from trio~=0.17->selenium) (25.1.0)
Requirement already satisfied: sortedcontainers in c:\data\python\lib\site-packages (from trio~=0.17->selenium) (2.4.0)
Requirement already satisfied: idna in c:\data\python\lib\site-packages (from trio~=0.17->selenium) (3.10)
Requirement already satisfied: outcome in c:\data\python\lib\site-packages (from trio~=0.17->selenium) (1.3.0.post0)
Requirement already satisfied: sniffio>=1.3.0 in c:\data\python\lib\site-packages (from trio~=0.17->selenium) (1.3.1)
Requirement already satisfied: cffi>=1.14 in c:\data\python\lib\site-packages (from trio~=0.17->selenium) (1.17.1)
Requirement already satisfied: wsproto>=0.14 in c:\data\python\lib\site-packages (from trio-websocket~=0.9->selenium) (1.2.0)
Requirement already satisfied: pysocks!=1.5.7,<2.0,>=1.5.6 in c:\data\python\lib\site-packages (from urllib3[socks]<3,>=1.26->selenium) (1.7.1)
Requirement already satisfied: charset-normalizer<4,>=2 in c:\data\python\lib\site-packages (from requests->webdriver-manager) (3.4.1)
Requirement already satisfied: pycparser in c:\data\python\lib\site-packages (from cffi>=1.14->trio~=0.17->selenium) (2.22)
Requirement already satisfied: h11<1,>=0.9.0 in c:\data\python\lib\site-packages (from wsproto>=0.14->trio-websocket~=0.9->selenium) (0.14.0)
Downloading webdriver_manager-4.0.2-py2.py3-none-any.whl (27 kB)
Downloading packaging-24.2-py3-none-any.whl (65 kB)
Downloading python_dotenv-1.0.1-py3-none-any.whl (19 kB)
Installing collected packages: python-dotenv, packaging, webdriver-manager
Successfully installed packaging-24.2 python-dotenv-1.0.1 webdriver-manager-4.0.2
[notice] A new release of pip is available: 24.3.1 -> 25.0.1
[notice] To update, run: python.exe -m pip install --upgrade pip
C:\Users\xxxxx>
pip install requests
実行すると・・・
C:\Users\xxxxx>pip install requests
Requirement already satisfied: requests in c:\data\python\lib\site-packages (2.32.3)
Requirement already satisfied: charset-normalizer<4,>=2 in c:\data\python\lib\site-packages (from requests) (3.4.1)
Requirement already satisfied: idna<4,>=2.5 in c:\data\python\lib\site-packages (from requests) (3.10)
Requirement already satisfied: urllib3<3,>=1.21.1 in c:\data\python\lib\site-packages (from requests) (2.3.0)
Requirement already satisfied: certifi>=2017.4.17 in c:\data\python\lib\site-packages (from requests) (2025.1.31)
[notice] A new release of pip is available: 24.3.1 -> 25.0.1
[notice] To update, run: python.exe -m pip install --upgrade pip
スクリプト
URLリストを読み込み、各URLのフルスクリーンショットを保存するPythonスクリプトです。
capture_screenshot.py
#20250616 改訂版 (c)sakaida.jp
import sys
import subprocess
import importlib
import shutil
import webbrowser
import time
import re
import os
import tkinter as tk
from tkinter import messagebox
# 必要なモジュールの一覧
required_modules = ["selenium", "requests", "packaging"]
# モジュールがなければインストールする
def install_missing_modules(modules):
for module in modules:
try:
importlib.import_module(module)
except ImportError:
print(f"{module} not found. Installing...")
subprocess.check_call([sys.executable, "-m", "pip", "install", module])
install_missing_modules(required_modules)
# 必要モジュールをインポート
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.chrome.options import Options
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.webdriver.common.alert import Alert
from selenium.common.exceptions import WebDriverException, TimeoutException
import requests
from packaging import version
# Chromeバージョン取得
def get_local_chrome_version():
try:
if sys.platform.startswith("win"):
import winreg
reg_path = r"SOFTWARE\Google\Chrome\BLBeacon"
key = winreg.OpenKey(winreg.HKEY_CURRENT_USER, reg_path)
value, _ = winreg.QueryValueEx(key, "version")
return value
elif sys.platform.startswith("darwin"):
result = subprocess.run(["/Applications/Google Chrome.app/Contents/MacOS/Google Chrome", "--version"], capture_output=True, text=True)
return result.stdout.strip().split()[-1]
else: # Linux
result = subprocess.run(["google-chrome", "--version"], capture_output=True, text=True)
return result.stdout.strip().split()[-1]
except Exception as e:
print(f"Could not determine Chrome version: {e}")
return None
# ChromeDriverバージョン取得
def get_chromedriver_version(driver_path):
try:
result = subprocess.run([driver_path, "--version"], capture_output=True, text=True)
match = re.search(r"ChromeDriver (\d+\.\d+\.\d+\.\d+)", result.stdout)
if match:
return match.group(1)
except Exception as e:
print(f"Could not determine ChromeDriver version: {e}")
return None
# バージョン整合性チェック
def check_version_match(chrome_version, chromedriver_version):
return chrome_version.split('.')[0] == chromedriver_version.split('.')[0]
# バージョンエラー時のダイアログとURL案内
def show_version_mismatch_dialog():
root = tk.Tk()
root.withdraw()
messagebox.showerror(
"ChromeDriverバージョンエラー",
"ChromeとChromeDriverのバージョンが一致していません。\n修正ページを開きます。"
)
webbrowser.open("https://googlechromelabs.github.io/chrome-for-testing/")
sys.exit(1)
# WebDriver初期化関数
def initialize_webdriver(webdriver_path, browser_width, headless=True):
chrome_options = Options()
if headless:
chrome_options.add_argument("--headless")
chrome_options.add_argument(f"--window-size={browser_width}x2160")
else:
chrome_options.add_argument("--start-maximized")
chrome_options.add_argument("--ignore-certificate-errors")
chrome_options.add_argument("--allow-running-insecure-content")
service = Service(webdriver_path)
return webdriver.Chrome(service=service, options=chrome_options)
# Basic認証処理
def handle_basic_auth(driver, basic_auth_id, basic_auth_pass):
try:
WebDriverWait(driver, 5).until(EC.alert_is_present())
alert = Alert(driver)
alert.send_keys(f"{basic_auth_id}\n{basic_auth_pass}")
alert.accept()
except TimeoutException:
print("認証ダイアログは表示されませんでした。")
# スクリーンショット処理
def capture_screenshot(driver, file_path, browser_width):
driver.set_window_size(browser_width, 2160)
WebDriverWait(driver, 10).until(lambda d: d.execute_script("return document.readyState") == "complete")
last_height = driver.execute_script("return Math.max(document.body.scrollHeight, document.documentElement.scrollHeight)")
while True:
driver.execute_script("window.scrollTo(0, document.body.scrollHeight);")
time.sleep(2)
new_height = driver.execute_script("return Math.max(document.body.scrollHeight, document.documentElement.scrollHeight)")
if new_height == last_height:
break
last_height = new_height
final_height = last_height + 300
driver.set_window_size(browser_width, final_height)
driver.execute_script("window.scrollTo(0, document.body.scrollHeight);")
time.sleep(1)
driver.save_screenshot(file_path)
# メイン処理
try:
print("引数:", sys.argv)
url = sys.argv[1]
file_path = sys.argv[2]
webdriver_path = sys.argv[3]
browser_width = int(sys.argv[4])
basic_auth_id = sys.argv[5] if len(sys.argv) > 5 else None
basic_auth_pass = sys.argv[6] if len(sys.argv) > 6 else None
if not url.startswith("http"):
url = "https://" + url
if basic_auth_id and basic_auth_pass:
protocol, host = url.split("://")
url = f"{protocol}://{basic_auth_id}:{basic_auth_pass}@{host}"
# ChromeとChromeDriverのバージョン取得
chrome_version = get_local_chrome_version()
chromedriver_version = get_chromedriver_version(webdriver_path)
print("Chrome:", chrome_version)
print("Driver:", chromedriver_version)
if not (chrome_version and chromedriver_version) or not check_version_match(chrome_version, chromedriver_version):
show_version_mismatch_dialog()
driver = initialize_webdriver(webdriver_path, browser_width)
try:
driver.get(url)
if basic_auth_id and basic_auth_pass:
handle_basic_auth(driver, basic_auth_id, basic_auth_pass)
WebDriverWait(driver, 5).until(EC.presence_of_element_located((By.TAG_NAME, 'body')))
capture_screenshot(driver, file_path, browser_width)
print("Saved to:", file_path)
finally:
driver.quit()
except IndexError:
print("Err:使い方: python script.py <url> <file_path> <webdriver_path> <browser_width> [<basic_auth_id> <basic_auth_pass>]")
except ValueError:
print("Err: browser_width は整数で指定してください。")
except Exception as e:
print(f"Err: 予期しないエラーが発生しました: {str(e)}")
#20250616 改訂版 (c)sakaida.jp
このスクリプトは、Seleniumを使用してWebページを開き、ページ全体をスクロールして完全にロードした後に、フルスクリーンショットを取得するものです。JavaScriptによる非同期描画や遅延読み込みにも対応しています。
主な機能:
- WebDriverの初期化
- ヘッドレスモード(GUIなし)で実行(ただし、要素の描画に影響する場合は無効化可能)。
- 初期ウィンドウサイズを
browser_width x 2160
に設定。 - 証明書エラーや安全でないコンテンツの実行許可。
- URLの整形とBasic認証対応
- コマンドライン引数で渡されたURLが
http://
やhttps://
を含まない場合、自動でhttps://
を付与。 - Basic認証情報がある場合、URLに埋め込むか、認証ダイアログを処理。
- コマンドライン引数で渡されたURLが
- ページの読み込み待機
document.readyState == "complete"
になるまで待機。- JavaScriptによる要素の描画が完了するまで、特定の要素の出現を待機。
- ページ全体のスクロール
- JavaScriptを使ってページの一番下までスクロールし、すべての要素をロード。
- フッターなどの遅延読み込み要素が完全に表示されるまで待機。
- スクロール完了後の処理
- 画面を先頭に戻す。
- 取得したページ全体の高さにウィンドウサイズを変更。
- スクリーンショットの取得
save_screenshot(file_path)
でページ全体のスクリーンショットを取得し、指定のパスに保存。
- エラーハンドリング
- WebDriver関連のエラー (
WebDriverException
やTimeoutException
) をキャッチしてエラーメッセージを出力。 - コマンドライン引数の不足や不正な値によるエラーを適切に処理。
- その他の予期しないエラーもキャッチし、エラーメッセージを出力。
- WebDriver関連のエラー (
使用方法:
python script.py <url> <file_path> <webdriver_path> <browser_width> [<basic_auth_id> <basic_auth_pass>]
<url>
: スクリーンショットを取得するページのURL<file_path>
: 画像ファイルの保存先<webdriver_path>
: Chrome WebDriverのパス<browser_width>
: ブラウザの横幅(例:1920
)[<basic_auth_id> <basic_auth_pass>]
: Basic認証が必要な場合に指定(オプション)
このスクリプトを使用することで、動的に描画されるページでも適切にスクリーンショットを取得できるようになります。